Node.js から MongoDB への接続テスト
結局、前記事(上記関連記事)の「The file system cache of this machine is configured to be greater than 40% of the total memory.」はまだ消えていません。とりあえず、Node.js からデータベースに接続してみようと思います。
まず、MongoDB を起動しておきます。ただ、まだ認証できるユーザを作っていませんので、WARNING を出したままで進みます。
>mongod --dbpath c:\mongodb\data
もうひとつコマンドプロンプトを立ち上げ、
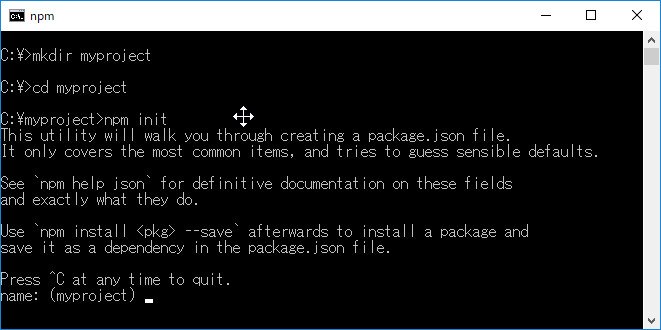
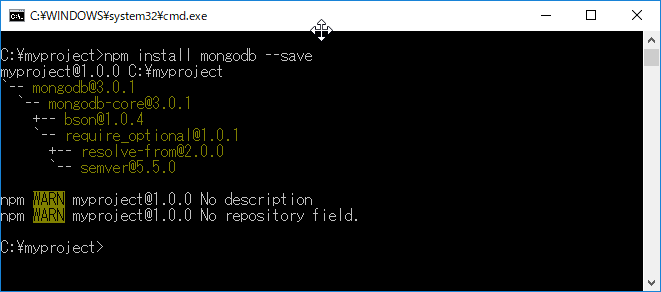
適当なフォルダをつくり、移動して npm init
で package.json をつくり、mongodb のドライバーモジュールをインストールします。
01MongoDB に接続
以下のチェック用スクリプトは、Quick Start にあります。
const MongoClient = require('mongodb').MongoClient; const assert = require('assert'); // Connection URL const url = 'mongodb://localhost:27017'; // Database Name const dbName = 'myproject'; // Use connect method to connect to the server MongoClient.connect(url, function(err, client) { assert.equal(null, err); console.log("Connected successfully to server"); const db = client.db(dbName); client.close(); });
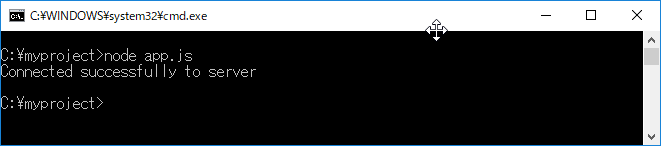
接続されています。
02ドキュメントの挿入
const insertDocuments = function(db, callback) { // Get the documents collection const collection = db.collection('documents'); // Insert some documents collection.insertMany([ {a : 1}, {a : 2}, {a : 3} ], function(err, result) { assert.equal(err, null); assert.equal(3, result.result.n); assert.equal(3, result.ops.length); console.log("Inserted 3 documents into the collection"); callback(result); }); } const MongoClient = require('mongodb').MongoClient; const assert = require('assert'); // Connection URL const url = 'mongodb://localhost:27017'; // Database Name const dbName = 'myproject'; // Use connect method to connect to the server MongoClient.connect(url, function(err, client) { assert.equal(null, err); console.log("Connected successfully to server"); const db = client.db(dbName); insertDocuments(db, function() { client.close(); }); });
3つのドキュメントを挿入する関数 insertDocument を書き加え呼び出します。
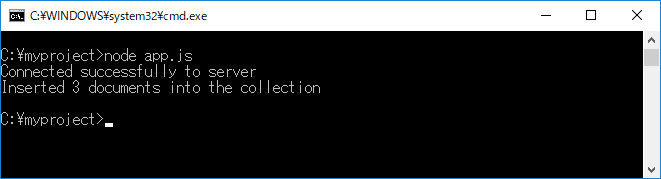
03ドキュメントの取得
const findDocuments = function(db, callback) { // Get the documents collection const collection = db.collection('documents'); // Find some documents collection.find({}).toArray(function(err, docs) { assert.equal(err, null); console.log("Found the following records"); console.log(docs) callback(docs); }); }
全てのドキュメントを取得する関数を書き加えます。
findDocuments(db, function() { client.close(); });
呼び出しのメソッドを書き換えます。
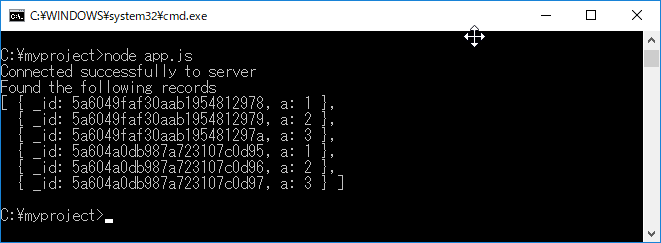
挿入を2回繰り返していますの6個のドキュメントが挿入されています。
といった感じで、リンク先の Quick Start にはドキュメントの選択取得、更新、削除、インデックス化のサンプルコードがあります。